The API Server can be called from anywhere by anything capable of making valid requests which meet the security requirements you've set up.
The most common areas you'll call workers from are in your forms, end points and workflow processes.
Working in End Points
When you're working in an end point you should use
Calling Another Worker
This example calls the iCM API worker to get back some information about an article. There's no need to provide an API key or server ID even though the target worker is internal.
function(params, credentials) {
let articleData = this.callWorkerMethod("icmapi", "CSArticle_get", {
"get": "ArticleHeading,ObjExtensionData",
"set": {
"ArticleID": 5877,
"ExtensionDataReqd": true,
"DetailLevel": 5
}
});
return articleData;
}
Calling Other End Points
It's worth noting that end points are themselves deployed to workers, and called as methods on those workers. If you are calling another end point deployed to the same worker as your current end point, use
This example calls another end point to return some configuration information.
function(params, credentials) {
let config = this.invokeEP("config.getConfig", {
"application": "TimsSolution"
});
return config.templateId;
}
Working in Forms
When working in forms you need to be aware of where your scripts are being executed, as this will restrict the API Server workers you can access.
The majority of your requests to the API Server will need to be made server-side, because most workers are only available to scripts executed on your server, preventing them from being called by a user's browser or an external service. That means using form field default functions and server-side variables.
In a standard installation the only workers you can call browser-side are the Ajax Library (which is where public end points are deployed), the Postcode worker (thanks to a framework proxy), and the Forms Service itself.
Browser-Side Calls
Apart from the Postcode worker, the only other requests you can make to the API Server in your form field handlers and page/form "init" functions are to end points deployed to the Ajax Library worker. These requests are made after the form has loaded and dynamically as field values are updated.
Take great care when writing an end point for the Ajax Library. They can be called by anyone, anywhere and are completely public.
You can construct requests using the .utilInvokeAPIServer helper function. There are also two form fields that can be used for browser-side calls.
Ajax Template
The HTML Ajax Template field calls an End Point and displays the result using a Handlebars template. There's an example in the Getting Started article.
Ajax Call
The API Server AJAX field (found in the "Misc" category of field types), can be set up to make an AJAX request whenever its value changes, whenever the value of another field changes, or when its "makeRequest" custom function is invoked.
In this example a request is made to an End Point, which is passed the value of another field as a parameter.
The field's handler then updates another field with the result of the call.
Server-Side Calls
A form field's default function is executed server-side when the form page it is on is being generated. The result of the function is returned to the field.
The helper function .utilServerAPIServerCall can be used to call an API Server worker. The function only works server-side and is able to make calls to internal workers as standard.
In this example the iCM API worker is asked for the heading of an article.
function(helper, defaultValue, currentValue) {
var request = helper.utilServerAPIServerCall("icmapi", "CSArticle_get", {
"get": "ArticleHeading",
"set": {
"ArticleID": 1
},
});
return request.ArticleHeading;
}
There are also blockly blocks you can use to call server-side end points and the Form Utils worker.
There's a lot more information about end points in Calling End Points From Forms.
Workflow Activities
The process modeller includes two activities that can make requests to the API Server. The workflow Activities article has more information on each of them.
Which you decide to use is up to you. The API Server task can communicate directly with any worker, but you'll need script activities either side of it to set up the parameters of your call and to process the "raw" result you get back. The End Point task only calls end points.
API Server Task
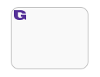
The API Server task can make a request to any worker. The properties let you set the worker to call, the method on the worker, and the name of a process variable that holds the relevant parameters. The result of the call is stored in the return variable, which can be given any suitable name.
End Point Task
The End Point task is similar to the API Server task, but has prompts to help you construct the request. The end point you want to call can be chosen from a pop-up menu and the parameters object can be populated from the schema or test cases defined in the end point itself.
From Somewhere Else
You can also make requests to the API Server from external applications. Postman (opens new window) is a free tool you can use to make requests and examine the responses.

If you have Postman you can copy the request in the image above and get results back from the Postcode worker on this website.
The basic structure to call a worker on the API Server is https://<subsiteURL>/apiserver/<worker-name>.
There's more information about external requests (although focused on end points) in Calling End Points Externally.