Grids help structure content into organised rows and columns, creating clean, responsive layouts that adapt seamlessly to different screen sizes.
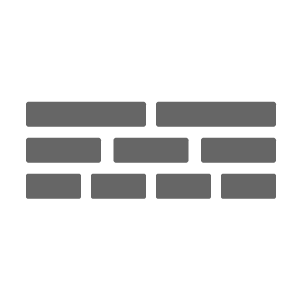
Grid components provide a flexible way to organise content into rows and columns, making it easy to create structured, responsive layouts. They help ensure content aligns neatly, maintains balance, and adapts seamlessly to different screen sizes. Additionally, grids offer control over spacing, alignment, and hierarchy, improving both design consistency and user experience.
When to use this component
Grid components are useful for creating organised layouts by dividing content into rows and columns. They work well for structuring complex pages, like dashboards or image galleries, where alignment and spacing are essential. Grids are also highly adaptable, allowing content to adjust smoothly to different screen sizes, ensuring a clear and consistent design across devices.
Examples in GOSS products
- The List template in all of its configurations
- The Default template is laid out differently for each theme using grids
Accessibility considerations
Wherever possible the items in a grid layout should be ordered visually in the same order that they appear in the DOM; we should never be trying to decouple the logical and visual order of the page. This is because screen-readers and keyboard navigation will not have the same experience as the visual order.
General notes
Grid and GridCell components that do not contain markup should always omit output of the structural elements, since empty structural elements are considered bad practice and should only occur in exceptional circumstances.
For our grid component, we have followed the trend of similar grid systems and accommodated up to 12 columns only.
Basic grid
A grid with no additional configuration options.
Max columns attribute
A grid with the grid max columns attribute set to '3'.
data-grid-maxcols="3"
The data-grid-maxcols attribute sets the maximum number of columns that the grid can have within its layout. In this case, with data-grid-maxcols="3", the grid will not exceed four columns, no matter how much space is available. This attribute is especially useful for ensuring the layout remains visually balanced and functional, even on larger screens or wider viewports.
<div data-grid-maxcols="3" class="grid grid--3col">
<div class="grid__cell">
<div class="grid__cellwrap">1 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">2 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">3 column</div>
</div>
</div>
<comp:grid cols="3">
<comp:gridCell>
1 column
</comp:gridCell>
<comp:gridCell>
2 column
</comp:gridCell>
<comp:gridCell>
3 column
</comp:gridCell>
</comp:grid>
@{
using (Html.Grid(new GridSettings()
{
Columns = 3,
MinColWidth = 1,
Step = 0,
Modifiers = new string[] { },
Datagrid = false
}
))
{
using (Html.GridCell(1, new string[] { }))
{
<p>1 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>2 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>3 column</p>
}
}
}
Split columns grid
A grid with the grid cell split columns attribute set to '2' and '1'.
data-grid-colspan="2"
The data-grid-colspan attribute controls how many columns a grid cell spans, giving you flexibility in designing layouts. For example, a cell with a colspan of 2 spans two columns, while one with a colspan of 1 uses a single column. This makes it easy to create varied layouts that adapt to the content's importance or size.
Using the attribute allows you to highlight key elements by giving them more space or fit smaller items neatly alongside larger ones. This customisation ensures a well-organised and visually appealing grid, even when combining different cell sizes in the same row.
<div data-grid-maxcols="3" class="grid grid--3col">
<div data-grid-colspan="2" class="grid__cell grid__cell--cols2">
<div class="grid__cellwrap">1 column (2/3)</div>
</div>
<div data-grid-colspan="1" class="grid__cell grid__cell--cols1">
<div class="grid__cellwrap">2 column (1/3)</div>
</div>
</div>
<comp:grid cols="3">
<comp:gridCell colspan="2">
1 column (2/3)
</comp:gridCell>
<comp:gridCell>
2 column (1/3)
</comp:gridCell>
</comp:grid>
@{
using (Html.Grid(new GridSettings()
{
Columns = 3,
MinColWidth = 1,
Step = 0,
Modifiers = new string[] { },
Datagrid = false
}
))
{
using (Html.GridCell(2, new string[] { }))
{
<p>1 column (2/3)</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>2 column(1/3)</p>
}
}
}
Responsive grid
A grid with the minimum column width attribute set to '250' and grid step attribute set to '2'.
data-grid-mincolwidth="250"
Setting the minimum column width attribute, controls the minimum width each column can have, ensuring the grid cells remain legible and functional. In this example, the minimum column width is 250 pixels. When the viewport becomes smaller, the grid will reduce the number of columns to maintain this minimum width.
data-grid-step="2"
By setting the grid step attribute, it defines how many columns are added or removed as the viewport changes size. A step of 2 means the grid will adjust by two columns at a time, creating smooth transitions between layouts as the screen resizes.
Together, these attributes allow the grid to adapt automatically to different screen sizes, ensuring that content is displayed in an organised and accessible way, whether on a large monitor or a smaller mobile device.
<div data-grid-maxcols="4" data-grid-mincolwidth="250" data-grid-step="2" class="grid grid--4col">
<div class="grid__cell">
<div class="grid__cellwrap">1 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">2 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">3 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">4 column</div>
</div>
</div>
<comp:grid cols="4" minColWidth="250" step="2">
<comp:gridCell>
1 column
</comp:gridCell>
<comp:gridCell>
2 column
</comp:gridCell>
<comp:gridCell>
3 column
</comp:gridCell>
<comp:gridCell>
4 column
</comp:gridCell>
</comp:grid>
@{
using (Html.Grid(new GridSettings()
{
Columns = 4,
MinColWidth = 250,
Step = 2,
Modifiers = new string[] { },
Datagrid = true
}
))
{
using (Html.GridCell(1, new string[] { }))
{
<p>1 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>2 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>3 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>4 column</p>
}
}
}
Grid within grid
Grids within grids allow for flexible layouts, where each section can have its own column structure tailored to its content. This makes the page feel organised and well-balanced.
They also support responsiveness, adjusting to different screen sizes. This ensures that content remains accessible and visually appealing on both mobile and desktop devices.
1 column (2/3)
2 column (1/3)
<div data-grid-maxcols="3" class="grid grid--3col">
<div data-grid-colspan="2" class="grid__cell grid__cell--cols2">
<div class="grid__cellwrap">
<h3>1 column (2/3)</h3>
<div data-grid-maxcols="4" data-grid-mincolwidth="50" data-grid-step="2" class="grid grid--4col">
<div class="grid__cell">
<div class="grid__cellwrap">1 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">2 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">3 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">4 column</div>
</div>
</div>
</div>
</div>
<div data-grid-colspan="1" class="grid__cell grid__cell--cols1">
<div class="grid__cellwrap">
<h3>2 column (1/3)</h3>
<div data-grid-maxcols="2" data-grid-mincolwidth="150" data-grid-step="1" class="grid grid--2col">
<div class="grid__cell">
<div class="grid__cellwrap">1 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">2 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">3 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">4 column</div>
</div>
</div>
</div>
</div>
</div>
<comp:grid cols="3">
<comp:gridCell colspan="2">
<h3>1 column (2/3)</h3>
<comp:grid cols="4" step="2" minColWidth="50">
<comp:gridCell>
1 column
</comp:gridCell>
<comp:gridCell>
2 column
</comp:gridCell>
<comp:gridCell>
3 column
</comp:gridCell>
<comp:gridCell>
4 column
</comp:gridCell>
</comp:grid>
</comp:gridCell>
<comp:gridCell>
<h3>2 column (1/3)</h3>
<comp:grid cols="2" step="1" minColWidth="150">
<comp:gridCell>
1 column
</comp:gridCell>
<comp:gridCell>
2 column
</comp:gridCell>
<comp:gridCell>
3 column
</comp:gridCell>
<comp:gridCell>
4 column
</comp:gridCell>
</comp:grid>
</comp:gridCell>
</comp:grid>
@{
using (Html.Grid(new GridSettings()
{
Columns = 3,
Datagrid = true
}
))
{
using (Html.GridCell(2, new string[] { }))
{
<h1>1 Column (2/3)</h1>
using (Html.Grid(new GridSettings()
{
Columns = 4,
MinColWidth = 50,
Step = 2
}))
{
using (Html.GridCell(1, new string[] { }))
{
<p>1 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>2 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>3 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>4 column</p>
}
}
<h1>2 Column (1/3)</h1>
using (Html.Grid(new GridSettings()
{
Columns = 2,
MinColWidth = 150,
Step = 1
}))
{
using (Html.GridCell(1, new string[] { }))
{
<p>1 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>2 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>3 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>4 column</p>
}
}
}
}
}
Grid modifiers
A grid with the center class applied and hide class applied to the last cell.
grid grid--center
The center class helps centre the grid content, making it look neat and aligned in the middle of the page. This is especially useful for keeping content balanced and visually pleasing, particularly on larger screens where elements can otherwise look scattered.
grid__cell grid__cell--hide
The hide cell class hides specific content on certain screen sizes or conditions. It's helpful for making a grid more responsive, allowing content to disappear when it's not needed or would clutter the layout. This keeps the page clean and focused on what's important.
<div data-grid-maxcols="3" class="grid grid--3col grid--center">
<div class="grid__cell">
<div class="grid__cellwrap">1 column</div>
</div>
<div class="grid__cell">
<div class="grid__cellwrap">2 column</div>
</div>
<div class="grid__cell grid__cell--hide">
<div class="grid__cellwrap">3 column</div>
</div>
</div>
<comp:grid cols="3" modifiers="center">
<comp:gridCell colspan="2">
1 column
</comp:gridCell>
<comp:gridCell>
2 column
</comp:gridCell>
<comp:gridCell modifiers="hide">
3 column
</comp:gridCell>
</comp:grid>
@{
using (Html.Grid(new GridSettings()
{
Columns = 3,
Modifiers = new string[] { "center" },
Datagrid = false
}
))
{
using (Html.GridCell(1, new string[] { }))
{
<p>1 column</p>
}
using (Html.GridCell(1, new string[] { }))
{
<p>2 column</p>
}
using (Html.GridCell(1, new string[] { "hide" }))
{
<p>3 column</p>
}
}
}