Diary Planner has three levels of configuration: global, application and appointment.
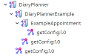
While there are certain settings which must be set at the appointment level, anything not set there will fall back to the application level. Anything not set at the application level will fall back to the global settings.
Appointment Configuration
function(params, credentials) {
//This is the id of the slot type article in this environment for this type of appointment
let appointmentTypeArticleId = 2098;
//Add any additional values that you want made available to CTM templates
let additionalCTMValues = {};
//Add any additional settings that you want made available to the appointment forms
let additionalSettings = {};
let taskAssignment = {};
let response = {
slotTypeId: appointmentTypeArticleId,
additionalCTMValues: additionalCTMValues,
additionalSettings: additionalSettings,
taskAssignment: taskAssignment,
showOnDashboard: true
};
return response;
}
Setting | Type | Description |
---|---|---|
appointmentTypeArticleId | Integer, required | This is the article ID of the Slot Type Template of this appointment type |
All remaining settings are optional. If not provided those set at the application or global level will be used. See below |
Global and Application Configuration
While these settings can be overridden at the appointment level, it doesn't generally make sense to.
Link Settings
The following settings must be correct for your platform, they are used by the booking forms to look up available appointments. They are also used to generate links that can be used in messages and emails.
let linkSettings = {
admin: {
subsite: "staff"
},
csu: {
subsite: "staff"
},
citizen: {
language: {
EN: {
subsite: "citizen"
}
}
}
};
Setting | Type | Description |
---|---|---|
role | Object | The roles defined for your user groups (see the roles section below) |
role.subsite | String | The name of the subsite the users in this role access (eg the name of your staff site for the staff user role) |
role.articles | Object | Article links to be output for this site, this can be picked up in your comms manager templates |
role.articles.<articleName> | String | Set <articleName> to the name for the article Set the value to the friendly url or the article id of the article |
role.language | Object | If you have different sites for multiple language support, the name of each subsite needs to be added to a language code object |
role.language.<languageCode> | Object | Set to the two digit language code that corresponds to the site's language |
role.language.<languageCode>.subsite | String | The name of the subsite |
role.language.<languageCode>.articles | Object | Article links to be output for this site |
role.language.<languageCode>.articles.<articleName> | String | Set <articleName> to the name for the article Set the value to the friendly url or the article id of the article |
User Task Settings
These setting control the forms and user groups used to manage and process the appointment.
The
let taskAssignment = {
default: {
managementForm: "SBDEFAULTMANAGEAPPOINTMENT",
processingForm: "SBDEFAULTPROCESSAPPOINTMENT",
confirmationForm: "SBDEFAULTCONFIRMATIONFORM"
},
csu: {
assignmentGroup: "CSA_GENERAL"
},
admin: {
managementForm: "SBCUSTOMFORM",
assignmentGroup: "SLOTBOOKINGADMIN"
}
};
Setting | Type | Description |
---|---|---|
default | Object | Default settings for the forms to be used |
managementForm | String | The name of the form to used to manage the appointment Default value - SBDEFAULTMANAGEAPPOINTMENT |
processingForm | String | The name of the form used by administrators for processing an appointment Default value - SBDEFAULTPROCESSAPPOINTMENT |
confirmationForm | String | The name of the form used to confirm the appointment Default value - SBDEFAULTCONFIRMATIONFORM |
csu | Object | Settings for csu user, any of the default settings can be overridden if required |
assignmentGroup | String | The name of the site user group for CSU tasks |
managementForm/processingForm/confirmationForm | String | Any forms you'd like to override |
admin | Object | Settings for csu user, any of the default settings can be overridden if required |
assignmentGroup | String | The name of the site user group for the initial administrator task |
managementForm/processingForm/confirmationForm | String | Any forms you'd like to override |
User Roles
These settings control the user roles available in the rest of the settings and the user groups that are associated with a role. When creating a new application, we recommend that you create a specific user group for administrators and update the admin user role to use that group.
Setting | Type | Description |
---|---|---|
anonymousUserId | Integer | The site user id of the anonymous user |
anonymousUserRole | String | The name of the anonymous user role in your application. It is highly recommended that you do not change this value |
roles | Array (Role Setting Objects) | An object for each of the user roles you'd like to define, by default there are three: admin - The administrators user group csu - The customer services user group citizen - The user group for users with a site user account who will be making bookings |
Role Settings
The following settings are used to define a user role.
Setting | Type | Description |
---|---|---|
code | String | The code for the user role |
name | String | The display name for the user role, this is for use in a future form-based configuration module |
siteUserGroups | Array (String) | The list of site user groups that are in this role |
isAdmin | Boolean | If true, this is the administration role |
isCSU | Boolean | If true, this is the CSU role |
canSelectAppointment | Boolean | If true, this user can use create appointments using the Appointment Selection Form when using the calendar |
Example
let userRoles = {
"anonymousUserRole": "anonymous",
"anonymousUserId": 1,
"roles": [{
"code": "admin",
"siteUserGroups": ["SLOTBOOKINGADMIN"],
"name": "Admin",
"isAdmin": true,
"isCSU": true,
"canSelectAppointments": true
}, {
"code": "csu",
"siteUserGroups": ["CSA_GENERAL"],
"name": "CSU",
"isAdmin": false,
"isCSU": true,
"canSelectAppointments": false
}, {
"code": "citizen",
"siteUserGroups": ["CITIZENS"],
"name": "Citizen",
"isAdmin": false,
"isCSU": false,
"canSelectAppointments": false
}]
};
Action Settings
The following settings control when, where and by whom actions can be performed. They are held in the variable
Setting | Type | Description |
---|---|---|
status | Object | The actions that will be available at each status |
processingActions | Object | The action processing settings by action |
Status Codes
The following are the default status codes for an appointment. They correspond to the steps in the appointment processing model. These can be extended if required for a new application:
Status | Description |
---|---|
CREATED | Initial status |
CHECKED_IN | Appointment has been checked in |
ASSIGNED | Appointment has been assigned but not started |
IN_PROGRESS | Appointment has been started |
COMPLETED | Appointment has been competed |
Action Availability Settings
These settings control when and by whom actions can be performed, the settings are stored against each status.
Setting | Type | Description |
---|---|---|
canCancel | Object | Whether the appointment can be cancelled at the current status |
canAmend | Object | Whether the appintment can be amended at the current status |
canReschedule | Object | Whether the appointment can be rescheduled at the current status |
canCheckin | Object | Whether the appointment can be checked in at the current status |
canReturn | Object | Whether the button to return to the calendar from the appointment form appears at the current status |
canUpdate | Object | Whether the updates to the appointment (like notes) can be made at the current status |
canAssign | Object | Whether the appointment can be assigned to a user at the current status |
canStart | Object | Whether the appointment can be started at the current status |
canComplete | Object | Whether the appointment can be completed at the current status |
For each of the above the following settings are available
Setting | Type | Description |
---|---|---|
always | Boolean | If true, the action can be performed by any administration user |
isAssigned | Boolean | If true, the action can only be performed if the administration task has been assigned and only by the assigned user |
userRoles | Array (String) | The list of user roles that can perform this action |
clearAssignment | Boolean | If true, automatically clears the assigned user when the action is performed |
Action Processing Settings
These settings control what happens when an action is performed, the behaviour for Cancel, Amend and Reschedule cannot be controlled via configuration. Those actions are performed by the Slot Booking process and cannot be altered.
Available settings for an action
Setting | Type | Description | ||||||
---|---|---|---|---|---|---|---|---|
autoAssign | Boolean | If true, when the action is performed the administration task will be automatically assigned to the current user | ||||||
nextForm | String | Sets the name of the form that will be used for the next administration task | ||||||
nextGroup | String | Sets the name of the candidate group for performing the next administration task | ||||||
nextDescription | String | Sets the description shown in self-service of the next administration task. The following replacement tokens can be inserted in the description.
| ||||||
nextStatus | String | Sets the status of the appointment after the action has been completed | ||||||
ticketStatus | String | Sets the status of the book and pay ticket that holds the appointment details | ||||||
clearForm | Boolean | If true, the administration form setting will be cleared. This should only be done if no further administration processing is required on the appointment | ||||||
clearUser | Boolean | If true, the assigned user for the administration task will be cleared. The task can then be claimed by any member of the candidate group for the task |
Additional Comms Template Manager Settings
This object lets you pass additional variables to the comms template manager to use in your email and message templates.
let additionalCTMValues = {
diaryPlanner: "DiaryPlanner"
};